You could flatten the two Arrays into one long Array, create two masks: one with 1s where there is a value in the first Array and 0s where there was a value in the second Array (and vice versa for the second mask). Then use those masks as probability distributions for the nextRandomIndexFromDistributions:distributionIndex: Capytalk message, with !Index selecting between your two original Arrays of values. This technique can be used on an arbitrary number of Arrays.
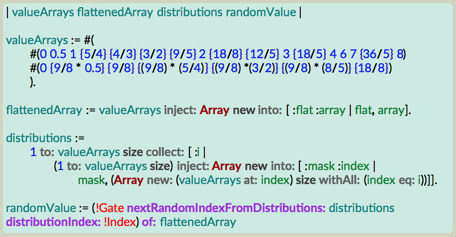
Here is the code for convenient copy/paste:
| valueArrays flattenedArray distributions randomValue |
valueArrays := #(
#(0 0.5 1 {5/4} {4/3} {3/2} {9/5} 2 {18/8} {12/5} 3 {18/5} 4 6 7 {36/5} 8)
#(0 {9/8 * 0.5} {9/8} {(9/8) * (5/4)} {(9/8) *(3/2)} {(9/8) * (8/5)} {18/8})
).
flattenedArray := valueArrays inject: Array new into: [ :flat :array | flat, array].
distributions :=
1 to: valueArrays size collect: [ :i |
(1 to: valueArrays size) inject: Array new into: [ :mask :index |
mask, (Array new: (valueArrays at: index) size withAll: (index eq: i))]].
randomValue := (!Gate nextRandomIndexFromDistributions: distributions distributionIndex: !Index) of: flattenedArray